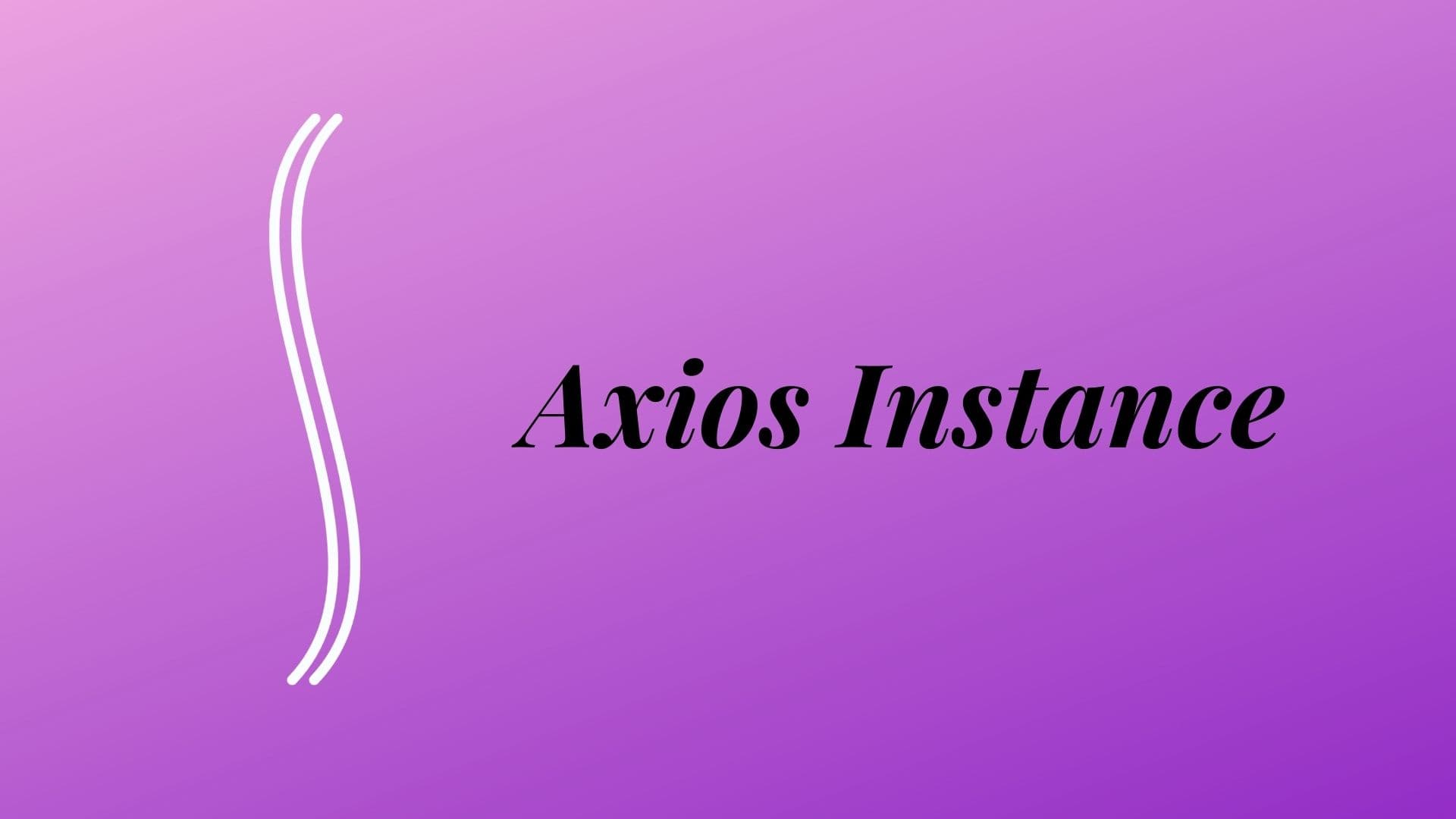
Create an Axios instance
7 min read
Prequisite:
- Should have good knowledge of Javascript and REST API
- At least know the basics of Axios
If you are into programming, then you will likely have heard of DRY (don' repeat yourself). Basically, writing the same code repeatedly can be quite tedious. And it becomes more tedious when trying to remember the url to make your Axios fetch a request every time. Would it not be great to just type in the url once, and code any methods you require out of it. Plus, you configure to any specific pathnames and query string. That's where the create() method comes in.
Axios provides a number of methods you can use, but create() is one of the most useful ones. It creates an instance Promise object of the url API.And you can use that instance Promise however many times you like and whichever methods you need.
Usually it is best to create a separate file just for the instance alone, and then we can import the instance to any file.
// ./apiInstance/jsonPlaceholder.js fileimport axios from "axios";
export default function jsonP_instance() {const baseUrl = "https://jsonplaceholder.typicode.com/users" const instance = axios.create({baseURL: baseUrl,timeout: 4000});return instance;};
Creating an instance is simple enough, you only need the url API and optionally set a timeout. Once done, we can now use it freely and however many times we want, without having to memorise the url again. The instance is a Promise object, so when we use it, we get the Resolved or Rejected response. Let's check out the example below:
import jsonP_instance from "./apiInstance/jsonPlaceholder.js";
// GET all users from Json Placeholder api// Same as GET "https://jsonplaceholder.typicode.com/users"jsonP_instance().get("/").then(response => console.log(response.data).catch(error => console.error(error.data);
// GET one user from Json Placeholder api// Same as GET "https://jsonplaceholder.typicode.com/users/1" jsonP_instance().get("/1").then(response => console.log(response.data).catch(error => console.error(error.data);
As you can see, the instance is easy to use, just like a normal fetch request promise object. And it is matched with its flexibility because, as mentioned before, you can use any methods you want (eg. POST, PUT, DELETE, etc) the same way as GET, you can configure the headers, insert a body, etc...
So if you have begun a project, knowing you are going to use the same url API over and over, Axios instance is definitely the way to go.